Controls the optional 128×64 pixel monochrome OLED display. More...
Files | |
file | display.h |
Functions | |
int8_t | sb_display_enable () |
Activate (and setup) the OLED-display. More... | |
int8_t | sb_display_available () |
Check if a functional OLED display is present. More... | |
int8_t | sb_display_disable () |
Turn the OLED display off. More... | |
int8_t | sb_display_draw (uint8_t pageNum, uint8_t colNum, uint8_t content) |
Draw a single page segment onto the OLED display. More... | |
int8_t | sb_display_drawBitmap (uint8_t pageStart, uint8_t colStart, uint8_t pageCount, uint8_t colCount, const uint8_t *contents) |
Draw a (rectangular) bitmap onto the OLED display. More... | |
int8_t | sb_display_fillScreen (const uint8_t *contents) |
Draw the contents of a 8*128 item 8-bit array (8 pages with 128 columns, each filled with 8 bit) onto the OLED Display. More... | |
int8_t | sb_display_showString (uint8_t pageNum, uint8_t colStart, const char *str) |
Print a \0 terminated text string on the OLED display using a 8×8 pixel font. More... | |
int8_t | sb_display_showStringWide (uint8_t pageNum, uint8_t colStart, const char *str) |
Print a \0 terminated text string with a (slightly) wider 8×8 pixel font onto the OLED display. More... | |
int8_t | sb_display_showStringSmall (uint8_t pageNum, uint8_t colStart, const char *str) |
Print a \0 terminated text string on the OLED display using a small 6×4 pixel font. More... | |
Detailed Description
Controls the optional 128×64 pixel monochrome OLED display.
The SPiCboard v3 has a TWI (two wire interface, also known as I²C), which can be used to connect an OLED display. Especially the SSD1306 monochrome OLED display fits perfectly onto the board. It has a 0.96 inch diameter with 128 horizontal and 64 vertical pixels.
For the sake of simplicity the horizontal pixels are termed columns. The vertical pixels are divided into 8 so-called pages, therefore a byte can represent the contents of a page segment (with 8 pixels - from the LSB on top to the MSB on bottom of each page segement).

For additional details please have a look into the SSD1306 Datasheet.
In case your run into memory issues (which is quite easy with only 2 KB SRAM) you might want to have a look into the FromFlash function variants.
- Warning
- The display is quite sensitive to fluctuation of current. Try to avoid contact of any [semi-]conductive material (including your fingers) with the pins on the Xplained Mini Board – especially connecting PC3 with PC4 – or the connection to your display might stop (it stalls until you restart it, the LED on the Xplained Mini Board can indicate the error).
Function Documentation
◆ sb_display_available()
int8_t sb_display_available | ( | ) |
Check if a functional OLED display is present.
Tries to connect to the SSD1306 display (I²C device address 0x78
) without enabling it.
- Return values
-
0 display is available and seems to work <0 display not available or not functional
◆ sb_display_disable()
int8_t sb_display_disable | ( | ) |
Turn the OLED display off.
- Return values
-
0 success <0 on I²C error (
- See also
- sb_display_enable() return codes)
◆ sb_display_draw()
int8_t sb_display_draw | ( | uint8_t | pageNum, |
uint8_t | colNum, | ||
uint8_t | content | ||
) |
Draw a single page segment onto the OLED display.
- Parameters
-
pageNum page number (0-7) for vertical positioning the output colNum column number (0-127) for the horizontal position of the output content contents to be drawn on the page segment (LSB on top and MSB on bottom)
- Return values
-
0 success <0 on I²C error (
- See also
- sb_display_enable() return codes)
◆ sb_display_drawBitmap()
int8_t sb_display_drawBitmap | ( | uint8_t | pageStart, |
uint8_t | colStart, | ||
uint8_t | pageCount, | ||
uint8_t | colCount, | ||
const uint8_t * | contents | ||
) |
Draw a (rectangular) bitmap onto the OLED display.
The result is similar to multiple calls of sb_display_draw() (although the execution of this function is way faster):
Example:
We want to draw a square with a point in its center with some spacing to the to the left top corner of the screen.
The bitmap will be drawn across two pages, each with eight page segments.
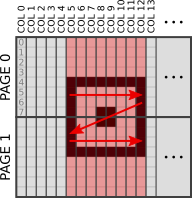
Hence we first initialize an array with 2*8 elements (columns 5-12 for page 0 and 1) containing the contents of each page segement (1 for a white pixel and 0 for a black/clear one) and then transfer them to the screen.
- Parameters
-
pageStart first page to set the top vertical position colStart first column to set the left horizontal position pageCount number of pages to set the bottom position (including the first page) colCount number of columns to define the right border (including the first column) contents array pointing to the bitmap (must have pageCount * colCount
elements) - orNULL
to clear the area
- Return values
-
0 success <0 on I²C error (see sb_display_enable() return codes)
◆ sb_display_enable()
int8_t sb_display_enable | ( | ) |
Activate (and setup) the OLED-display.
- Return values
-
0 success -1 initialization of I²C failed -2 setting I²C device address failed -3 writing to I²C device failed -4 timeout during I²C communication -5 out of bounds (addressing nonexistent page/column)
◆ sb_display_fillScreen()
int8_t sb_display_fillScreen | ( | const uint8_t * | contents | ) |
Draw the contents of a 8*128
item 8-bit array (8 pages with 128 columns, each filled with 8 bit) onto the OLED Display.
The function call is identical to
In case the pointer is NULL
, the entire screen will be cleared.
- Warning
- Make sure that the buffer points to an array with 1024 items - this is C, there is no range check!
- Parameters
-
contents pointer to a 8*128
item array - or NULL to clear the screen
- Return values
-
0 success <0 on I²C error (
- See also
- sb_display_enable() return codes)
◆ sb_display_showString()
int8_t sb_display_showString | ( | uint8_t | pageNum, |
uint8_t | colStart, | ||
const char * | str | ||
) |
Print a \0
terminated text string on the OLED display using a 8×8 pixel font.
A single character will fit into one page (= eight rows) and eight columns. Therefore it is possible to print 16 characters at maximum.
If the length of the string exceeds the available columns (= columns right of the start column) in the current page, the output will not be continued on the next line!
The font was extract from the Linux kernel and is based on code page 437 (= extended ASCII, every possible character has a graphical string representation).
Consequently, the ASCII control characters (like line break) are displayed with special symbols but don't have any functional impact.
The Fonts Table contains a detailed list of the available characters
- Warning
- The font will be stored on the flash which will consume about 2300 bytes.
- Parameters
-
pageNum page number (0-7) for vertical positioning the output colStart first column to set the horizontal position of the output str 0-terminated string
- Return values
-
>=0 length of printed string (can be less than strlen(str) ) <0 on I²C error (
- See also
- sb_display_enable() return codes)
◆ sb_display_showStringSmall()
int8_t sb_display_showStringSmall | ( | uint8_t | pageNum, |
uint8_t | colStart, | ||
const char * | str | ||
) |
Print a \0 terminated text string on the OLED display using a small 6×4 pixel font.
A single character will fit into one page (eight rows, even though it is just six pixels) and four columns, therefore it is possible to print 32 characters at maximum.
If the length of the string exceeds the available columns (= columns right of the start column) in the current page, the output will not be continued on the next line!
The Fonts Table contains a detailed list of the available characters
- Warning
- In contrast to the other
showString
functions the character set is quite limited - it only supports printable ASCII characters (neither control characters nor the extended ASCII set). Hence, the required flash memory consumption is (with about 700 bytes) less than a third of the space requirements of the other fonts.
- Parameters
-
pageNum page number (0-7) for vertical positioning the output colStart first column to set the horizontal position of the output str 0-terminated string
- Return values
-
>=0 length of printed string (can be less than strlen(str) ) <0 on I²C error (
- See also
- sb_display_enable() return codes)
◆ sb_display_showStringWide()
int8_t sb_display_showStringWide | ( | uint8_t | pageNum, |
uint8_t | colStart, | ||
const char * | str | ||
) |
Print a \0
terminated text string with a (slightly) wider 8×8 pixel font onto the OLED display.
The character set is almost identical to the sb_display_showString() function and the space requirement is the same (up to 16 characters per line)
The Fonts Table contains a detailed list of the available characters.
- Warning
- This font will consume about 2300 bytes, too.
- Parameters
-
pageNum page number (0-7) for vertical positioning the output colStart first column to set the horizontal position of the output str 0-terminated string
- Return values
-
>=0 length of printed string (can be less than strlen(str) ) <0 on I²C error (see sb_display_enable() return codes)